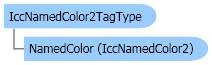
Visual Basic (Declaration) | |
---|---|
Public Class IccNamedColor2TagType Inherits IccTagTypeBase |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As IccNamedColor2TagType |
C# | |
---|---|
public class IccNamedColor2TagType : IccTagTypeBase |
C++/CLI | |
---|---|
public ref class IccNamedColor2TagType : public IccTagTypeBase |
This example method can be used in creating an "namedColor2Type" mentioned in the ICC.1:2004-10 specification.
Visual Basic | ![]() |
---|---|
Public Sub IccNamedColor2TagTypeExample() ' load an Icc Profile Dim iccProfile As New IccProfileExtended(Path.Combine(LEAD_VARS.ImagesDir, "EmptyIcc.icc")) ' define the color root names Dim colorRootName1(31) As Byte colorRootName1(0) = CByte(AscW("a"c)) colorRootName1(1) = CByte(AscW("n"c)) colorRootName1(2) = CByte(AscW("y"c)) colorRootName1(3) = CByte(AscW(" "c)) colorRootName1(4) = CByte(AscW("n"c)) colorRootName1(5) = CByte(AscW("a"c)) colorRootName1(6) = CByte(AscW("m"c)) colorRootName1(7) = CByte(AscW("e"c)) colorRootName1(8) = CByte(AscW("1"c)) colorRootName1(9) = 0 Dim colorRootName2(31) As Byte colorRootName2(0) = CByte(AscW("a"c)) colorRootName2(1) = CByte(AscW("n"c)) colorRootName2(2) = CByte(AscW("y"c)) colorRootName2(3) = CByte(AscW(" "c)) colorRootName2(4) = CByte(AscW("n"c)) colorRootName2(5) = CByte(AscW("a"c)) colorRootName2(6) = CByte(AscW("m"c)) colorRootName2(7) = CByte(AscW("e"c)) colorRootName2(8) = CByte(AscW("2"c)) colorRootName2(9) = 0 ' define color PCS coordinates, must be 3 values only Dim colorPCSCoords() As UShort = {1, 2, 3} ' and then define the Device coordinates Dim colorDEVCoords() As UShort = {4, 3, 2, 1} ' define two named color data Dim namedColors() As IccNamedColor2Data = { _ New IccNamedColor2Data(colorRootName1, colorPCSCoords, colorDEVCoords), _ New IccNamedColor2Data(colorRootName2, colorPCSCoords, colorDEVCoords)} ' any vendor flag Dim vendorFlag As Integer = &H1234 ' the prefix for each color name, must be 32 bytes Dim prefix(31) As Byte prefix(0) = CByte(AscW("a"c)) prefix(1) = CByte(AscW("n"c)) prefix(2) = CByte(AscW("y"c)) prefix(3) = CByte(AscW(" "c)) prefix(4) = CByte(AscW("p"c)) prefix(5) = CByte(AscW("r"c)) prefix(6) = CByte(AscW("e"c)) prefix(7) = CByte(AscW("f"c)) prefix(8) = CByte(AscW("i"c)) prefix(9) = CByte(AscW("x"c)) prefix(10) = 0 ' the suffix for each color name, must be 32 bytes Dim suffix(31) As Byte suffix(0) = CByte(AscW("a"c)) suffix(1) = CByte(AscW("n"c)) suffix(2) = CByte(AscW("y"c)) suffix(3) = CByte(AscW(" "c)) suffix(4) = CByte(AscW("s"c)) suffix(5) = CByte(AscW("u"c)) suffix(6) = CByte(AscW("f"c)) suffix(7) = CByte(AscW("f"c)) suffix(8) = CByte(AscW("i"c)) suffix(9) = CByte(AscW("x"c)) suffix(10) = 0 ' create the IccNamedColor2 class Dim namedColor2 As New IccNamedColor2(vendorFlag, prefix, suffix, namedColors) ' define the tag type Dim namedColor2TagType As New IccNamedColor2TagType(namedColor2) ' add the new tag to the ICC Profile iccProfile.AddTag(namedColor2TagType, IccTag.NamedColor2Tag, IccTagTypeBase.NamedColor2TypeSignature) ' generate the new profile id iccProfile.GenerateProfileId() ' update the icc array with the new changes iccProfile.UpdateDataArray() ' write the Icc Profile into a new file iccProfile.GenerateIccFile(Path.Combine(LEAD_VARS.ImagesDir, "IccNamedColor2TagTypeVB.icc")) End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void IccNamedColor2TagTypeExample() { // load an Icc Profile string fileName = Path.Combine(LEAD_VARS.ImagesDir, "EmptyIcc.icc"); IccProfileExtended iccProfile = new IccProfileExtended(fileName); // define the color root names byte[] colorRootName1 = new byte[32]; colorRootName1[0] = (byte)'a'; colorRootName1[1] = (byte)'n'; colorRootName1[2] = (byte)'y'; colorRootName1[3] = (byte)' '; colorRootName1[4] = (byte)'n'; colorRootName1[5] = (byte)'a'; colorRootName1[6] = (byte)'m'; colorRootName1[7] = (byte)'e'; colorRootName1[8] = (byte)'1'; colorRootName1[9] = (byte)0; byte[] colorRootName2 = new byte[32]; colorRootName2[0] = (byte)'a'; colorRootName2[1] = (byte)'n'; colorRootName2[2] = (byte)'y'; colorRootName2[3] = (byte)' '; colorRootName2[4] = (byte)'n'; colorRootName2[5] = (byte)'a'; colorRootName2[6] = (byte)'m'; colorRootName2[7] = (byte)'e'; colorRootName2[8] = (byte)'2'; colorRootName2[9] = (byte)0; // define color PCS coordinates, must be 3 values only ushort[] colorPCSCoords = new ushort[3] { 1, 2, 3 }; // and then define the Device coordinates ushort[] colorDEVCoords = new ushort[4] { 4, 3, 2, 1 }; // define two named color data IccNamedColor2Data[] namedColors = new IccNamedColor2Data[2]; namedColors[0] = new IccNamedColor2Data(colorRootName1, colorPCSCoords, colorDEVCoords); namedColors[1] = new IccNamedColor2Data(colorRootName2, colorPCSCoords, colorDEVCoords); // any vendor flag int vendorFlag = 0x00001234; // the prefix for each color name, must be 32 bytes byte[] prefix = new byte[32]; prefix[0] = (byte)'a'; prefix[1] = (byte)'n'; prefix[2] = (byte)'y'; prefix[3] = (byte)' '; prefix[4] = (byte)'p'; prefix[5] = (byte)'r'; prefix[6] = (byte)'e'; prefix[7] = (byte)'f'; prefix[8] = (byte)'i'; prefix[9] = (byte)'x'; prefix[10] = (byte)0; // the suffix for each color name, must be 32 bytes byte[] suffix = new byte[32]; suffix[0] = (byte)'a'; suffix[1] = (byte)'n'; suffix[2] = (byte)'y'; suffix[3] = (byte)' '; suffix[4] = (byte)'s'; suffix[5] = (byte)'u'; suffix[6] = (byte)'f'; suffix[7] = (byte)'f'; suffix[8] = (byte)'i'; suffix[9] = (byte)'x'; suffix[10] = (byte)0; // create the IccNamedColor2 class IccNamedColor2 iccNamedColor2 = new IccNamedColor2(vendorFlag, prefix, suffix, namedColors); // define the tag type IccNamedColor2TagType namedColor2TagType = new IccNamedColor2TagType(iccNamedColor2); // add the new tag to the ICC Profile iccProfile.AddTag(namedColor2TagType, IccTag.NamedColor2Tag, IccTagTypeBase.NamedColor2TypeSignature); // generate the new profile id iccProfile.GenerateProfileId(); // update the icc array with the new changes iccProfile.UpdateDataArray(); // write the Icc Profile into a new file string IccfileName = Path.Combine(LEAD_VARS.ImagesDir, "IccNamedColor2TagTypeCS.icc"); iccProfile.GenerateIccFile(IccfileName); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
SilverlightVB | ![]() |
---|---|
- The signature for this tag type is IccTagTypeBase.NamedColor2TypeSignature.
System.Object
Leadtools.ColorConversion.IccTagTypeBase
Leadtools.ColorConversion.IccNamedColor2TagType
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
IccNamedColor2TagType MembersLeadtools.ColorConversion Namespace
IccTagTypeBase Class
IccCurveTagType Class
IccDateTimeTagType Class
IccDataTagType Class
IccLookupTable16TagType Class
IccLookupTable8TagType Class
IccMeasurementTagType Class
IccParametricCurveTagType Class
IccResponseCurveSet16TagType Class
IccViewingConditionsTagType Class
IccChromaticityTagType Class
IccColorantTableTagType Class
IccMultiLocalizedUnicodeTagType Class
IccColorantOrderTagType Class
IccLookupTableAToBTagType Class
IccLookupTableBToATagType Class
IccProfileSequenceDescriptionTagType Class
IccS15Fixed16ArrayTagType Class
IccSignatureTagType Class
IccTextTagType Class
IccU16Fixed16ArrayTagType Class
IccUint16ArrayTagType Class
IccUint32ArrayTagType Class
IccUint64ArrayTagType Class
IccUint8ArrayTagType Class
IccXyzTagType Class
IccUnknownTagType Class
IccTools Class
IccProfileExtended Class