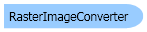
Visual Basic (Declaration) | |
---|---|
Public MustInherit NotInheritable Class RasterImageConverter |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As RasterImageConverter |
C# | |
---|---|
public static class RasterImageConverter |
C++/CLI | |
---|---|
public ref class RasterImageConverter abstract sealed |
Visual Basic | ![]() |
---|---|
Public Sub RasterImageConverterExample() Dim codecs As New RasterCodecs() Dim srcFileName As String = Path.Combine(LEAD_VARS.ImagesDir, "Image1.cmp") Dim destFileName1 As String = Path.Combine(LEAD_VARS.ImagesDir, "GdiPlusImage.bmp") Dim destFileName2 As String = Path.Combine(LEAD_VARS.ImagesDir, "RasterImage.bmp") ' Load a RasterImage, convert to GDI+ image Using leadImage As RasterImage = codecs.Load(srcFileName) ' Convert to GDI+ image Using gdipImage As Image = RasterImageConverter.ConvertToImage(leadImage, ConvertToImageOptions.None) ' Save it to disk gdipImage.Save(destFileName1, ImageFormat.Bmp) End Using End Using ' Load a GDI+ image, convert to RasterImage Using gdipImage As Image = Image.FromFile(destFileName1) ' Convert to RasterImage Using leadImage As RasterImage = RasterImageConverter.ConvertFromImage(gdipImage, ConvertFromImageOptions.None) ' Save it to disk codecs.Save(leadImage, destFileName2, RasterImageFormat.Bmp, 0) End Using End Using ' Clean up codecs.Dispose() End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void RasterImageConverterExample() { RasterCodecs codecs = new RasterCodecs(); string srcFileName = Path.Combine(LEAD_VARS.ImagesDir, "Image1.cmp"); string destFileName1 = Path.Combine(LEAD_VARS.ImagesDir,"GdiPlusImage.bmp"); string destFileName2 = Path.Combine(LEAD_VARS.ImagesDir, "RasterImage.bmp"); // Load a RasterImage, convert to GDI+ image using(RasterImage leadImage = codecs.Load(srcFileName)) { // Convert to GDI+ image using(Image gdipImage = RasterImageConverter.ConvertToImage(leadImage, ConvertToImageOptions.None)) { // Save it to disk gdipImage.Save(destFileName1, ImageFormat.Bmp); } } // Load a GDI+ image, convert to RasterImage using(Image gdipImage = Image.FromFile(destFileName1)) { // Convert to RasterImage using(RasterImage leadImage = RasterImageConverter.ConvertFromImage(gdipImage, ConvertFromImageOptions.None)) { // Save it to disk codecs.Save(leadImage, destFileName2, RasterImageFormat.Bmp, 0); } } // Clean up codecs.Dispose(); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
The LEADTOOLS RasterImage class provides platform independent representation of an image. It serves as a working area for image manipulation and conversion. LEADTOOLS functions use this class for accessing the image in memory and for maintaining the characteristics of the image. This class contains the functionality needed to convert a LEADTOOLS Leadtools.RasterImage to and from a Windows GDI or GDI+ image objects.
The RasterImageConverter class contains the following functionality:
Method | Description |
---|---|
ConvertToImage |
Converts a LEADTOOLS Leadtools.RasterImage to GDI+ System.Drawing.Image |
ConvertFromImage |
Converts a GDI+ System.Drawing.Image to LEADTOOLS Leadtools.RasterImage |
ChangeToImage |
Converts a LEADTOOLS Leadtools.RasterImage to GDI+ System.Drawing.Image sharing the same memory. |
MakeCompatible |
Makes a Leadtools.RasterImage pixel and color format compatible with GDI+ |
TestCompatible, GetNearestBitsPerPixel, IsValidBitsPerPixel and GetNearestPixelFormat |
Utility methods for testing and making a LEADTOOLS Leadtools.RasterImage GDI+ compatible |
FromHBitmap and ToHBitmap |
Converts a Leadtools.RasterImage to and from a GDI DDB (Device Dependant Bitmap) |
ChangeFromHBitmap and ChangeToHBitmap |
Converts a Leadtools.RasterImage to and from a GDI DDB (Device Dependant Bitmap) while sharing the same memory |
ToWmf, FromWmf, ToEmf and FromEmf |
Converts a Leadtools.RasterImage to and from a Windows Metafile or Enhanced Metafile |
ChangeToWmf, ChangeFromWmf, ChangeToEmf and ChangeFromEmf |
Converts a Leadtools.RasterImage to and from a Windows Metafile or Enhanced Metafile while sharing the same memory |
For more information refer to RasterImage and GDI/GDI+.
System.Object
Leadtools.Drawing.RasterImageConverter
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)