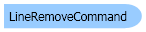
Visual Basic (Declaration) | |
---|---|
Public Class LineRemoveCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As LineRemoveCommand |
C# | |
---|---|
public class LineRemoveCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class LineRemoveCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Run the LineRemoveCommand on an image.
Visual Basic | ![]() |
---|---|
Public WithEvents lineRemoveCommand_S1 As LineRemoveCommand Public Sub LineRemoveCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Clean.tif")) ' Prepare the command lineRemoveCommand_S1 = New LineRemoveCommand lineRemoveCommand_S1.Type = LineRemoveCommandType.Horizontal lineRemoveCommand_S1.Flags = LineRemoveCommandFlags.UseGap lineRemoveCommand_S1.GapLength = 2 lineRemoveCommand_S1.MaximumLineWidth = 5 lineRemoveCommand_S1.MinimumLineLength = 200 lineRemoveCommand_S1.MaximumWallPercent = 10 lineRemoveCommand_S1.Wall = 7 lineRemoveCommand_S1.Run(leadImage) End Sub Private Sub LineRemoveCommand_LineRemove_S1(ByVal sender As Object, ByVal e As LineRemoveCommandEventArgs) Handles lineRemoveCommand_S1.LineRemove MessageBox.Show("Row Col " + "( " + e.StartRow.ToString() + ", " + e.StartColumn.ToString() + " )" + _ Chr(13) + " Length " + e.Length.ToString()) e.Status = RemoveStatus.Remove End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void LineRemoveCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Clean.tif")); // Prepare the command LineRemoveCommand command = new LineRemoveCommand(); command.LineRemove += new EventHandler<LineRemoveCommandEventArgs>(LineRemoveEvent_S1); command.Type = LineRemoveCommandType.Horizontal; command.Flags = LineRemoveCommandFlags.UseGap; command.GapLength = 2; command.MaximumLineWidth = 5; command.MinimumLineLength = 200; command.MaximumWallPercent = 10; command.Wall = 7; command.Run(image); } private void LineRemoveEvent_S1(object sender, LineRemoveCommandEventArgs e) { MessageBox.Show("Row Col " + "( " + e.StartRow.ToString() + ", " + e.StartColumn + " )" + "\n Length " + e.Length.ToString()); e.Status= RemoveStatus.Remove; } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
public void LineRemoveCommandExample(RasterImage image, Stream outStream) { // Prepare the command LineRemoveCommand command = new LineRemoveCommand(); command.LineRemove += new EventHandler<LineRemoveCommandEventArgs>(LineRemoveEvent_S1); command.Type = LineRemoveCommandType.Horizontal; command.Flags = LineRemoveCommandFlags.UseGap; command.GapLength = 2; command.MaximumLineWidth = 5; command.MinimumLineLength = 200; command.MaximumWallPercent = 10; command.Wall = 7; command.Run(image); // Save result image RasterCodecs codecs = new RasterCodecs(); codecs.Save(image, outStream, RasterImageFormat.CcittGroup4, 1); image.Dispose(); } private void LineRemoveEvent_S1(object sender, LineRemoveCommandEventArgs e) { Debug.WriteLine("Row Col " + "( " + e.StartRow.ToString() + ", " + e.StartColumn + " )" + "\n Length " + e.Length.ToString()); e.Status= RemoveStatus.Remove; } |
SilverlightVB | ![]() |
---|---|
Public Sub LineRemoveCommandExample(ByVal image As RasterImage, ByVal outStream As Stream) ' Prepare the command Dim command As LineRemoveCommand = New LineRemoveCommand() AddHandler command.LineRemove, AddressOf LineRemoveEvent_S1 command.Type = LineRemoveCommandType.Horizontal command.Flags = LineRemoveCommandFlags.UseGap command.GapLength = 2 command.MaximumLineWidth = 5 command.MinimumLineLength = 200 command.MaximumWallPercent = 10 command.Wall = 7 command.Run(image) ' Save result image Dim codecs As RasterCodecs = New RasterCodecs() codecs.Save(image, outStream, RasterImageFormat.CcittGroup4, 1) image.Dispose() End Sub Private Sub LineRemoveEvent_S1(ByVal sender As Object, ByVal e As LineRemoveCommandEventArgs) Debug.WriteLine("Row Col " & "( " & e.StartRow.ToString() & ", " & e.StartColumn & " )" & Constants.vbLf & " Length " & e.Length.ToString()) e.Status= RemoveStatus.Remove End Sub |
- This command removes horizontal and vertical lines from scanned text documents. If the lines pass through text, the LineRemoveCommand properties/Constructor parameters can be configured to remove or preserve the text. The behavior of this command can be further modified by using an Event Handler that handles the LineRemoveCommandEventArgs.
- This command works only on 1-bit black and white images.
- If a region is selected, only the selected region will be changed by this command. If no region is selected, the whole image will be processed.
- This command does not support signed data images.
- This command does not support 32-bit grayscale images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Core.LineRemoveCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
LineRemoveCommand MembersLeadtools.ImageProcessing.Core Namespace
Cleaning Up 1-Bit Images
SmoothCommand Class
BorderRemoveCommand Class
InvertedTextCommand Class
InvertedPageCommand Class
DotRemoveCommand Class
HolePunchRemoveCommand Class
HighQualityRotateCommand Class
MinimumCommand Class
MaximumCommand Class
Leadtools.ImageProcessing.Effects.RegionHolesRemovalCommand