The JpipServer Class includes members that are available as an add-on to the LEADTOOLS Imaging Pro, Document, and Medical Imaging toolkits.
Provides a simple, programmatically controlled JPIP protocol server.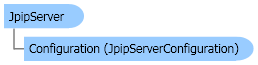
Visual Basic (Declaration) | |
---|---|
Public Class JpipServer |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As JpipServer |
C# | |
---|---|
public class JpipServer |
C++/CLI | |
---|---|
public ref class JpipServer |
Visual Basic | ![]() |
---|---|
Public Class RunServerExample Private _server As JpipServer Private _logWriter As WindowsLogWriter Private _responseCount As Integer Private _loggingFileName As String Private _loggingFileEnabled As Boolean Private Const LOCAL_IP_ADDRESS As String = "127.0.0.1" Private Const SERVER_NAME As String = "LEAD JPIP Server - Test Server" Private Const PORT_107 As Integer = 107 Private CACHE_DIRECTORY As String = Path.Combine(LEAD_VARS.ImagesDir, "jpeg2000") Private IMAGES_DIRECTORY As String = Path.Combine(LEAD_VARS.ImagesDir, "jpeg2000") Private IMAGE_NAME As String = Path.Combine(LEAD_VARS.ImagesDir, "Earth8000_Precint_4_.j2k") Public Sub New() Leadtools.Examples.Support.Unlock() _server = New JpipServer() _logWriter = New WindowsLogWriter() _responseCount = 0 _loggingFileEnabled = True _loggingFileName = Path.Combine(LEAD_VARS.ImagesDir, "Server Logs\log.txt") End Sub Public Sub StartServer() ResetServerConfiguration() 'Interactive handling AddHandler _server.RequestReceived, AddressOf _server_RequestReceived AddHandler _server.ResponseSending, AddressOf _server_ResponseSending 'Handle event logs AddHandler EventLogger.EventLog, AddressOf EventLogger_EventLog 'clear the server cache _server.ClearCacheContents() 'Add custom logging channel If (Not EventLogger.ContainsLoggingChannel(_logWriter)) Then EventLogger.AddLoggingChannel(_logWriter) End If EventLogger.LogEvent(SERVER_NAME, LOCAL_IP_ADDRESS, PORT_107.ToString(), "", "", DateTime.Now, Leadtools.Jpip.Logging.EventLogEntryEventType.Information, "", "LEAD Example Started") 'start the server _server.Start() Debug.Assert(_server.IsRunning, "Server did not start.") ' client side Dim jpipViewer As JpipRasterImageViewer = New JpipRasterImageViewer() SetViewer(jpipViewer) AddHandler jpipViewer.FileOpened, AddressOf jpipViewer_FileOpened jpipViewer.Open(IMAGE_NAME) End Sub Private Sub jpipViewer_FileOpened(ByVal sender As Object, ByVal e As EventArgs) Dim jpipViewer As JpipRasterImageViewer = DirectCast(sender, JpipRasterImageViewer) jpipViewer.ZoomIn() jpipViewer.Close() 'Server side If _server.IsRunning Then _server.Stop() End If EventLogger.LogEvent(SERVER_NAME, LOCAL_IP_ADDRESS, PORT_107.ToString(), "", "", DateTime.Now, Leadtools.Jpip.Logging.EventLogEntryEventType.Information, "", "LEAD Example Ended") End Sub Private Sub EventLogger_EventLog(ByVal sender As Object, ByVal e As Leadtools.Jpip.Logging.EventLogEntry) Console.WriteLine("Server Name: {0}; Server IP: {1}; Server Port: {2}; Client IP: {3}; Client Port: {4}; Channel ID: {5}; Event Type: {6}; Description: {7}", e.ServerName, e.ServerIPAddress, e.ServerPort, e.ClientIPAddress, e.ClientPort, e.ChannelID, e.Type.ToString(), e.Description) End Sub Private Sub _server_ResponseSending(ByVal sender As Object, ByVal e As ResponseSendingEventArgs) Dim dataFolder As String Dim dumpClientIP As String Dim dumpClientPort As Integer dataFolder = Path.Combine(LEAD_VARS.ImagesDir, "ServerResponses") dumpClientIP = "127.0.0.1" dumpClientPort = 121 If (Not Directory.Exists(dataFolder)) Then Directory.CreateDirectory(dataFolder) End If If (e.ClientIpAddress = dumpClientIP) AndAlso (e.ClientPort = dumpClientPort) Then Dim subDirectoryPath As String Dim responseDataFile As String _responseCount += 1 subDirectoryPath = String.Concat(dataFolder, "\", dumpClientIP) responseDataFile = String.Concat(subDirectoryPath, "\response_", _responseCount) If (Not Directory.Exists(subDirectoryPath)) Then Directory.CreateDirectory(subDirectoryPath) End If File.WriteAllBytes(responseDataFile, e.Data) End If End Sub Private Sub _server_RequestReceived(ByVal sender As Object, ByVal e As RequestReceivedEventArgs) Dim denyClientIpAddress As String = "127.0.0.1" Dim denyClientPort As Integer = 120 If (e.ClientIpAddress = denyClientIpAddress) AndAlso (e.ClientPort = denyClientPort) Then e.Deny = True e.StatusCode = CInt(HttpStatusCode.Forbidden) e.Description = "Server refused to process request." End If End Sub Public Sub ResetServerConfiguration() If (Not _server.IsRunning) Then Dim appConfigSettings As JpipConfiguration 'for reading from configuration file settings appConfigSettings = New JpipConfiguration() 'Server Settings _server.Configuration.ServerName = SERVER_NAME _server.Configuration.IPAddress = LOCAL_IP_ADDRESS _server.Configuration.Port = PORT_107 _server.Configuration.ImagesFolder = IMAGES_DIRECTORY _server.Configuration.CacheFolder = CACHE_DIRECTORY _server.Configuration.MaxServerBandwidth = ConfigurationDefaults.MaxServerBandwidth _server.Configuration.CacheSize = appConfigSettings.CacheSize _server.Configuration.DivideSuperBoxes = True _server.Configuration.ChunkSize = 512 _server.Configuration.MaxClientCount = 5 _server.Configuration.ConnectionIdleTimeout = TimeSpan.FromSeconds(ConfigurationDefaults.ConnectionIdleTimeout) _server.Configuration.MaxSessionLifetime = TimeSpan.FromSeconds(ConfigurationDefaults.MaxSessionLifetime) _server.Configuration.MaxConnectionBandwidth = ConfigurationDefaults.MaxConnectionBandwidth 'Communication Settings _server.Configuration.MaxTransportConnections = 15 _server.Configuration.HandshakeTimeout = TimeSpan.FromSeconds(ConfigurationDefaults.HandshakeTimeout) _server.Configuration.RequestTimeout = TimeSpan.FromSeconds(ConfigurationDefaults.RequestTimeout) _server.Configuration.ChunkSize = ConfigurationDefaults.ChunkSize 'Images Settings _server.Configuration.ImageParsingTimeout = TimeSpan.FromSeconds(ConfigurationDefaults.ImageParsingTimeout) _server.Configuration.PartitionBoxSize = 30 _server.Configuration.DivideSuperBoxes = appConfigSettings.DivideSuperBoxes 'Logging Settings _server.Configuration.LoggingFile = _loggingFileName _server.Configuration.EnableLogging = _loggingFileEnabled _server.Configuration.LogInformation = True _server.Configuration.LogWarnings = True _server.Configuration.LogDebug = True _server.Configuration.LogErrors = True AddAliasFolders() End If End Sub Private Sub AddAliasFolders() Dim alias_Renamed As String = "LeadImages" Dim physicalPath As String = LEAD_VARS.ImagesDir 'Set server aliases folder If _server.Configuration.IsAliasExists(alias_Renamed) Then If _server.Configuration.GetAliasPath(alias_Renamed) <> physicalPath Then _server.Configuration.RemoveAliasFolder(alias_Renamed) Else Return End If End If _server.Configuration.AddAliasFolder(alias_Renamed, physicalPath) End Sub Public Sub SetViewer(ByVal viewer As JpipRasterImageViewer) viewer.CacheDirectoryName = CACHE_DIRECTORY viewer.PortNumber = PORT_107 viewer.IPAddress = LOCAL_IP_ADDRESS viewer.PacketSize = 16384 viewer.ChannelType = JpipChannelTypes.HttpChannel End Sub Private Class WindowsLogWriter : Implements ILogginChannel Public Sub WriteLog(ByVal logEntry As Leadtools.Jpip.Logging.EventLogEntry) Implements ILogginChannel.WriteLog Dim message As String message = String.Format("Server Name: {0}; Server IP: {1}; Server Port: {2}; Client IP: {3}; Client Port: {4}; Channel ID: {5}; Event Type: {6}; Description: {7}", logEntry.ServerName, logEntry.ServerIPAddress, logEntry.ServerPort, logEntry.ClientIPAddress, logEntry.ClientPort, logEntry.ChannelID, logEntry.Type.ToString(), logEntry.Description) System.Diagnostics.EventLog.WriteEntry("JPIP LEAD Server", message) End Sub End Class End Class Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
private JpipServer _server; private WindowsLogWriter _logWriter; private int _responseCount; private string _loggingFileName; private bool _loggingFileEnabled; private const string LOCAL_IP_ADDRESS = "127.0.0.1"; private const string SERVER_NAME = "LEAD JPIP Server - Test Server"; private const int PORT_107 = 107; private string CACHE_DIRECTORY = Path.Combine(LEAD_VARS.ImagesDir, "jpeg2000"); private string IMAGES_DIRECTORY = Path.Combine(LEAD_VARS.ImagesDir, "jpeg2000"); private string IMAGE_NAME = Path.Combine(LEAD_VARS.ImagesDir, "Earth8000_Precint_4_.j2k"); private string LOG_DIRECTORY = Path.Combine(LEAD_VARS.ImagesDir, "Server Logs"); private string LOG_FILE_NAME = Path.Combine(LEAD_VARS.ImagesDir, "log.txt"); public RunServerExamples () { Leadtools.Examples.Support.Unlock(); _server = new JpipServer ( ) ; _logWriter = new WindowsLogWriter ( ) ; _responseCount = 0 ; _loggingFileEnabled = true; if(!File.Exists(LOG_DIRECTORY + LOG_FILE_NAME)) { Directory.CreateDirectory(LOG_DIRECTORY); File.WriteAllText(LOG_FILE_NAME, " "); } _loggingFileName = LOG_FILE_NAME; } void EventLogger_EventLog(object sender, Leadtools.Jpip.Logging.EventLogEntry e) { Console.WriteLine ( "Server Name: {0}; Server IP: {1}; Server Port: {2}; Client IP: {3}; Client Port: {4}; Channel ID: {5}; Event Type: {6}; Description: {7}", e.ServerName, e.ServerIPAddress, e.ServerPort, e.ClientIPAddress, e.ClientPort, e.ChannelID, e.Type.ToString ( ), e.Description ) ; } void _server_ResponseSending(object sender, ResponseSendingEventArgs e) { string dataFolder; string dumpClientIP ; int dumpClientPort ; dataFolder = Path.Combine(LEAD_VARS.ImagesDir, "ServerResponses"); dumpClientIP = "127.0.0.1" ; dumpClientPort = 1207 ; if (!Directory.Exists(dataFolder)) { Directory.CreateDirectory( dataFolder); } if ( (e.ClientIpAddress == dumpClientIP) && ( e.ClientPort == dumpClientPort ) ) { string subDirectoryPath; string responseDataFile; _responseCount++; subDirectoryPath = string.Concat(dataFolder, "\\", dumpClientIP); responseDataFile = string.Concat(subDirectoryPath, "\\response_", _responseCount); if (!Directory.Exists(subDirectoryPath)) { Directory.CreateDirectory(subDirectoryPath); } File.WriteAllBytes(responseDataFile, e.Data); } } void _server_RequestReceived(object sender, RequestReceivedEventArgs e) { string denyClientIpAddress = "127.0.0.1" ; int denyClientPort = 120 ; if ( ( e.ClientIpAddress == denyClientIpAddress ) && ( e.ClientPort == denyClientPort ) ) { e.Deny = true ; e.StatusCode = (int) HttpStatusCode.Forbidden; e.Description = "Server refused to process request."; } } public void ResetServerConfiguration ( ) { if ( !_server.IsRunning ) { JpipConfiguration appConfigSettings ; //for reading from configuration file settings appConfigSettings = new JpipConfiguration ( ) ; //Server Settings _server.Configuration.ServerName = SERVER_NAME; _server.Configuration.IPAddress = LOCAL_IP_ADDRESS; _server.Configuration.Port = PORT_107; _server.Configuration.ImagesFolder = IMAGES_DIRECTORY; _server.Configuration.CacheFolder = CACHE_DIRECTORY; _server.Configuration.MaxServerBandwidth = ConfigurationDefaults.MaxServerBandwidth; _server.Configuration.CacheSize = appConfigSettings.CacheSize; _server.Configuration.DivideSuperBoxes = true; _server.Configuration.ChunkSize = 512; _server.Configuration.MaxClientCount = 5; _server.Configuration.ConnectionIdleTimeout = TimeSpan.FromSeconds ( ConfigurationDefaults.ConnectionIdleTimeout ) ; _server.Configuration.MaxSessionLifetime = TimeSpan.FromSeconds ( ConfigurationDefaults.MaxSessionLifetime ) ; _server.Configuration.MaxConnectionBandwidth = ConfigurationDefaults.MaxConnectionBandwidth; //Communication Settings _server.Configuration.MaxTransportConnections = 15; _server.Configuration.HandshakeTimeout = TimeSpan.FromSeconds ( ConfigurationDefaults.HandshakeTimeout ) ; _server.Configuration.RequestTimeout = TimeSpan.FromSeconds ( ConfigurationDefaults.RequestTimeout ) ; _server.Configuration.ChunkSize = ConfigurationDefaults.ChunkSize; //Images Settings _server.Configuration.ImageParsingTimeout = TimeSpan.FromSeconds ( ConfigurationDefaults.ImageParsingTimeout ) ; _server.Configuration.PartitionBoxSize = 30; _server.Configuration.DivideSuperBoxes = appConfigSettings.DivideSuperBoxes; //Logging Settings _server.Configuration.LoggingFile = _loggingFileName; _server.Configuration.EnableLogging = _loggingFileEnabled; _server.Configuration.LogInformation = true; _server.Configuration.LogWarnings = true; _server.Configuration.LogDebug = true; _server.Configuration.LogErrors = true; AddAliasFolders(); } } private void AddAliasFolders() { string alias = "LeadImages"; string physicalPath = LEAD_VARS.ImagesDir; //Set server aliases folder if (_server.Configuration.IsAliasExists(alias)) { if (_server.Configuration.GetAliasPath(alias) != physicalPath) { _server.Configuration.RemoveAliasFolder(alias); } else { return ; } } _server.Configuration.AddAliasFolder(alias, physicalPath); } public void SetViewer(JpipRasterImageViewer viewer) { viewer.CacheDirectoryName = CACHE_DIRECTORY; viewer.PortNumber = PORT_107; viewer.IPAddress = LOCAL_IP_ADDRESS; viewer.PacketSize = 16384; viewer.ChannelType = JpipChannelTypes.HttpChannel; } private class WindowsLogWriter : ILogginChannel { public void WriteLog(Leadtools.Jpip.Logging.EventLogEntry logEntry) { string message; message = string.Format("Server Name: {0}; Server IP: {1}; Server Port: {2}; Client IP: {3}; Client Port: {4}; Channel ID: {5}; Event Type: {6}; Description: {7}", logEntry.ServerName, logEntry.ServerIPAddress, logEntry.ServerPort, logEntry.ClientIPAddress, logEntry.ClientPort, logEntry.ChannelID, logEntry.Type.ToString(), logEntry.Description); System.Diagnostics.EventLog.WriteEntry("JPIP LEAD Server", message); } } public void StartServer() { ResetServerConfiguration(); //Interactive handling _server.RequestReceived += new EventHandler<RequestReceivedEventArgs>(_server_RequestReceived); _server.ResponseSending += new EventHandler<ResponseSendingEventArgs>(_server_ResponseSending); //Handle event logs EventLogger.EventLog += new EventHandler<Leadtools.Jpip.Logging.EventLogEntry>(EventLogger_EventLog); //clear the server cache _server.ClearCacheContents(); //Add custom logging channel if (!EventLogger.ContainsLoggingChannel(_logWriter)) { EventLogger.AddLoggingChannel(_logWriter); } EventLogger.LogEvent(SERVER_NAME, LOCAL_IP_ADDRESS, PORT_107.ToString(), "", "", DateTime.Now, Leadtools.Jpip.Logging.EventLogEntryEventType.Information, "", "LEAD Example Started"); //start the server _server.Start(); Debug.Assert(_server.IsRunning, "Server did not start."); /* client side */ JpipRasterImageViewer jpipViewer = new JpipRasterImageViewer(); jpipViewer.FileOpened += new EventHandler(jpipViewer_FileOpened); SetViewer(jpipViewer); jpipViewer.Open(IMAGE_NAME); } void jpipViewer_FileOpened(object sender, EventArgs e) { JpipRasterImageViewer jpipViewer = ( JpipRasterImageViewer ) sender ; jpipViewer.ZoomIn(); jpipViewer.Close(); /*Server side*/ if (_server.IsRunning) { _server.Stop(); } EventLogger.LogEvent(SERVER_NAME, LOCAL_IP_ADDRESS, PORT_107.ToString(), "", "", DateTime.Now, Leadtools.Jpip.Logging.EventLogEntryEventType.Information, "", "LEAD Example Ended"); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
Using the Leadtools.Jpip.Server.JpipServer class, you can create a JPIP protocol server that responds to JPIP requests from any JPIP compliant client.
To start the server, create a Leadtools.Jpip.Server.JpipServer object then set the Configuration values and call the Start method, the Leadtools.Jpip.Server.JpipServer will listen to requests in a background threads causing the application main thread not to block.
When you are done with the Leadtools.Jpip.Server.JpipServer object call the Stop method.
System.Object
Leadtools.Jpip.Server.JpipServer
Target Platforms: Microsoft .NET Framework 2.0, Windows 2000, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7