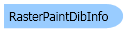
Visual Basic (Declaration) | |
---|---|
Public Class RasterPaintDibInfo |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As RasterPaintDibInfo |
C# | |
---|---|
public class RasterPaintDibInfo |
C++/CLI | |
---|---|
public ref class RasterPaintDibInfo |
This example will show how to indicate that the graphics device expects a 16-bit image with the image data in the low 12 bits. Also, this particular card expected the data to be top-down, unlike the usual GDI functions which expect the data to be upside-down.
The example is in C++/CLI because this is the most useful language for implementing these classes.
C# | ![]() |
---|---|
// macro that calculates the number of bytes per line, rounding up to a multiple of 4 bytes #define DIB_WIDTH_BYTES(pixels) ((((pixels) + 31) & ~31) >> 3) Object^ myPaintCallbacks::GetDibInfoCallback(RasterImage^ image, array<Object^>^ args) { // make sure the parameters are correct if(args->Length != 2 || image == nullptr) { throw gcnew RasterException(RasterExceptionCode::InvalidParameter); return nullptr; } UInt32 uWidth = (UInt32)args[1]; RasterPaintDibInfo^ pDibInfo = gcnew RasterPaintDibInfo; if(pDibInfo == nullptr) { throw gcnew RasterException(RasterExceptionCode::NoMemory); return nullptr; } pDibInfo->Default(); pDibInfo->BitsPerPixel = 16; // paint using 16-bit data, although there are only 12 significant bits pDibInfo->PlaneCount = 1; // One plane pDibInfo->BytesPerLine = DIB_WIDTH_BYTES(uWidth * 16); // bytes per line is a multiple of 4 bytes pDibInfo->ViewPerspective = RasterViewPerspective::TopLeft; // the data should be TOP_LEFT, not BOTTOM_LEFT like the data in the regular GDI functions pDibInfo->Order = RasterByteOrder::Gray; // gray bitmap // indicate that the data should be in the low 12 bits pDibInfo->Flags = RasterPaintDibInfoFlags::LowHighBitValid; pDibInfo->LowBit = 0; pDibInfo->HighBit = 11; return pDibInfo; } |
The user will typically allocate this class and return it from the RasterImagePaintCallbackFunction.GetDibInfoCallback callback.
System.Object
Leadtools.Drawing.RasterPaintDibInfo
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)