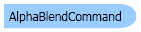
Visual Basic (Declaration) | |
---|---|
Public Class AlphaBlendCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As AlphaBlendCommand |
C# | |
---|---|
public class AlphaBlendCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class AlphaBlendCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Run the AlphaBlendCommand on an image.
Visual Basic | ![]() |
---|---|
Public Sub AlphaBlendCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")) ' Prepare the command Dim SrcImage As RasterImage SrcImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "ImageProcessingDemo\image3.cmp"), 4, CodecsLoadByteOrder.Bgr, 1, 1) Dim command As AlphaBlendCommand = New AlphaBlendCommand 'Combine SrcImage with image, with half opacity. command.DestinationRectangle = New LeadRect(leadImage.Width \ 8, leadImage.Height \ 8, leadImage.Width, leadImage.Height) command.SourceImage = SrcImage command.Opacity = 128 command.Run(leadImage) codecs.Save(leadImage, Path.Combine(LEAD_VARS.ImagesDir, "Result.jpg"), RasterImageFormat.Jpeg, 24) End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void AlphaBlendCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")); // Prepare the command RasterImage SrcImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, @"ImageProcessingDemo\Image3.cmp"), 4, CodecsLoadByteOrder.Bgr, 1, 1); AlphaBlendCommand command = new AlphaBlendCommand(); //Combine SrcImage with image, with half opacity. command.DestinationRectangle = new LeadRect(image.Width / 8, image.Height / 8, image.Width, image.Height); command.SourceImage = SrcImage; command.Opacity = 128; command.Run(image); codecs.Save(image, Path.Combine(LEAD_VARS.ImagesDir, "Result.jpg"), RasterImageFormat.Jpeg, 24); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
public void AlphaBlendCommandExample(RasterImage image, RasterImage srcImage, Stream outStream) { // Prepare the command AlphaBlendCommand command = new AlphaBlendCommand(); //Combine SrcImage with image, with half opacity. command.DestinationRectangle = new LeadRect(image.Width / 8, image.Height / 8, image.Width, image.Height); command.SourceImage = srcImage; command.Opacity = 128; command.Run(image); // Save result image RasterCodecs codecs = new RasterCodecs(); codecs.Save(image, outStream, RasterImageFormat.Jpeg, 24); image.Dispose(); srcImage.Dispose(); } |
SilverlightVB | ![]() |
---|---|
Public Sub AlphaBlendCommandExample(ByVal image As RasterImage, ByVal srcImage As RasterImage, ByVal outStream As Stream) ' Prepare the command Dim command As AlphaBlendCommand = New AlphaBlendCommand() 'Combine SrcImage with image, with half opacity. command.DestinationRectangle = New LeadRect(image.Width / 8, image.Height / 8, image.Width, image.Height) command.SourceImage = srcImage command.Opacity = 128 command.Run(image) ' Save result image Dim codecs As RasterCodecs = New RasterCodecs() codecs.Save(image, outStream, RasterImageFormat.Jpeg, 24) image.Dispose() srcImage.Dispose() End Sub |
- The opacity determines how much the affected image will show through the source image. For example, if the Opacity property (or the opacity parameter in the constructor) is set to 255 (completely opaque) then the source image will appear and the affected image will not show through the source image at all. If the opacity is 128 (half opacity), then the pixels for that area will consist of 50 percent from the source image and 50 percent from the affected image.
- This command supports 12 and 16-bit grayscale and 48 and 64-bit color images. Support for 12 and 16-bit grayscale and 48 and 64-bit color images is available only in the Document/Medical toolkits.
- This command does not support signed data images.
- This command does not support 32-bit grayscale images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Effects.AlphaBlendCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
AlphaBlendCommand MembersLeadtools.ImageProcessing.Effects Namespace
Introduction to Image Processing With LEADTOOLS
CombineCommand Class
FeatherAlphaBlendCommand Class
TextureAlphaBlendCommand Class
Leadtools.ImageProcessing.SpecialEffects.BricksTextureCommand
Leadtools.ImageProcessing.SpecialEffects.CanvasCommand
DisplacementCommand Class
Leadtools.ImageProcessing.SpecialEffects.FragmentCommand
Leadtools.ImageProcessing.SpecialEffects.VignnetCommand