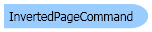
Visual Basic (Declaration) | |
---|---|
Public Class InvertedPageCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As InvertedPageCommand |
C# | |
---|---|
public class InvertedPageCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class InvertedPageCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
This example will show how to use the InvertedPageCommand class to check and auto-correct inverted images.
Visual Basic | ![]() |
---|---|
Public Sub InvertedPageCommandExample() Dim codecs As New RasterCodecs() ' Get an image Dim tifFileName As String = Path.Combine(LEAD_VARS.ImagesDir, "ocr1.tif") Dim invertedImageFileName As String = Path.Combine(LEAD_VARS.ImagesDir, "ocr1_Inverted.tif") Dim nonInvertedImageFileName As String = Path.Combine(LEAD_VARS.ImagesDir, "ocr1_NonInverted.tif") Dim image As RasterImage = codecs.Load(tifFileName) ' The images should be non-inverted at the beginning, check Dim invertedPage As New InvertedPageCommand(InvertedPageCommandFlags.NoProcess) invertedPage.Run(image) Console.WriteLine("Original image, inverted = {0}", invertedPage.IsInverted) ' Invert the image Dim invert As New InvertCommand() invert.Run(image) codecs.Save(image, invertedImageFileName, image.OriginalFormat, image.BitsPerPixel) ' Check again invertedPage.Run(image) Console.WriteLine("After running InvertCommand, inverted = {0}", invertedPage.IsInverted) ' Now run the command to un-invert the image invertedPage.Flags = InvertedPageCommandFlags.Process invertedPage.Run(image) ' Now check the image again invertedPage.Flags = InvertedPageCommandFlags.NoProcess invertedPage.Run(image) Console.WriteLine("After running InvertedPageCommand, inverted = {0}", invertedPage.IsInverted) codecs.Save(image, nonInvertedImageFileName, image.OriginalFormat, image.BitsPerPixel) image.Dispose() codecs.Dispose() End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void InvertedPageCommandExample() { RasterCodecs codecs = new RasterCodecs(); // Get an image string tifFileName = Path.Combine(LEAD_VARS.ImagesDir, "ocr1.tif"); string invertedImageFileName = Path.Combine(LEAD_VARS.ImagesDir, "ocr1_Inverted.tif"); string nonInvertedImageFileName = Path.Combine(LEAD_VARS.ImagesDir, "ocr1_NonInverted.tif"); RasterImage image = codecs.Load(tifFileName); // The images should be non-inverted at the beginning, check InvertedPageCommand invertedPage = new InvertedPageCommand(InvertedPageCommandFlags.NoProcess); invertedPage.Run(image); Console.WriteLine("Original image, inverted = {0}", invertedPage.IsInverted); // Invert the image InvertCommand invert = new InvertCommand(); invert.Run(image); codecs.Save(image, invertedImageFileName, image.OriginalFormat, image.BitsPerPixel); // Check again invertedPage.Run(image); Console.WriteLine("After running InvertCommand, inverted = {0}", invertedPage.IsInverted); // Now run the command to un-invert the image invertedPage.Flags = InvertedPageCommandFlags.Process; invertedPage.Run(image); // Now check the image again invertedPage.Flags = InvertedPageCommandFlags.NoProcess; invertedPage.Run(image); Console.WriteLine("After running InvertedPageCommand, inverted = {0}", invertedPage.IsInverted); codecs.Save(image, nonInvertedImageFileName, image.OriginalFormat, image.BitsPerPixel); image.Dispose(); codecs.Dispose(); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
public void InvertedPageCommandExample(RasterImage image, Stream outStream1, Stream outStream2) { // The images should be non-inverted at the beginning, check InvertedPageCommand invertedPage = new InvertedPageCommand(InvertedPageCommandFlags.NoProcess); invertedPage.Run(image); Debug.WriteLine("Original image, inverted = {0}", invertedPage.IsInverted); // Invert the image InvertCommand invert = new InvertCommand(); invert.Run(image); RasterCodecs codecs = new RasterCodecs(); // save image to "ocr1_Inverted.tif"; codecs.Save(image, outStream1, image.OriginalFormat, image.BitsPerPixel); // Check again invertedPage.Run(image); Debug.WriteLine("After running InvertCommand, inverted = {0}", invertedPage.IsInverted); // Now run the command to un-invert the image invertedPage.Flags = InvertedPageCommandFlags.Process; invertedPage.Run(image); // Now check the image again invertedPage.Flags = InvertedPageCommandFlags.NoProcess; invertedPage.Run(image); Debug.WriteLine("After running InvertedPageCommand, inverted = {0}", invertedPage.IsInverted); // save result image to "ocr1_NonInverted.tif" codecs.Save(image, outStream2, RasterImageFormat.CcittGroup4, 1); image.Dispose(); } |
SilverlightVB | ![]() |
---|---|
Public Sub InvertedPageCommandExample(ByVal image As RasterImage, ByVal outStream1 As Stream, ByVal outStream2 As Stream) ' The images should be non-inverted at the beginning, check Dim invertedPage As InvertedPageCommand = New InvertedPageCommand(InvertedPageCommandFlags.NoProcess) invertedPage.Run(image) Debug.WriteLine("Original image, inverted = {0}", invertedPage.IsInverted) ' Invert the image Dim invert As InvertCommand = New InvertCommand() invert.Run(image) Dim codecs As RasterCodecs = New RasterCodecs() ' save image to "ocr1_Inverted.tif"; codecs.Save(image, outStream1, image.OriginalFormat, image.BitsPerPixel) ' Check again invertedPage.Run(image) Debug.WriteLine("After running InvertCommand, inverted = {0}", invertedPage.IsInverted) ' Now run the command to un-invert the image invertedPage.Flags = InvertedPageCommandFlags.Process invertedPage.Run(image) ' Now check the image again invertedPage.Flags = InvertedPageCommandFlags.NoProcess invertedPage.Run(image) Debug.WriteLine("After running InvertedPageCommand, inverted = {0}", invertedPage.IsInverted) ' save result image to "ocr1_NonInverted.tif" codecs.Save(image, outStream2, RasterImageFormat.CcittGroup4, 1) image.Dispose() End Sub |
An inverted Leadtools.RasterImage is an image that has text or other objects in high contrast color on a low contrast background, for example white text on black background. This command can check whether an image is inverted as well as auto-correct it.
This command can only detect an entire image. To search for and correct specific inverted areas or regions in an image, use the InvertedTextCommand.
A common source of inverted images are black and white images saved with a non-standard palette by some applications.
This command does not support signed data images.
For more information, refer to Cleaning Up 1-Bit Images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Core.InvertedPageCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
InvertedPageCommand MembersLeadtools.ImageProcessing.Core Namespace
Cleaning Up 1-Bit Images
SmoothCommand Class
BorderRemoveCommand Class
LineRemoveCommand Class
InvertedTextCommand Class
HighQualityRotateCommand Class
DotRemoveCommand Class
HolePunchRemoveCommand Class
HighQualityRotateCommand Class
MinimumCommand Class
MaximumCommand Class
Leadtools.ImageProcessing.Effects.RegionHolesRemovalCommand