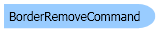
Visual Basic (Declaration) | |
---|---|
Public Class BorderRemoveCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As BorderRemoveCommand |
C# | |
---|---|
public class BorderRemoveCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class BorderRemoveCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Run the BorderRemoveCommand on an image.
Visual Basic | ![]() |
---|---|
Public WithEvents borderRemoveCommandCallback As BorderRemoveCommand Public Sub BorderRemoveCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Clean.tif")) ' Prepare the command borderRemoveCommandCallback = New BorderRemoveCommand borderRemoveCommandCallback.Border = BorderRemoveBorderFlags.All borderRemoveCommandCallback.Flags = BorderRemoveCommandFlags.UseVariance borderRemoveCommandCallback.Percent = 20 borderRemoveCommandCallback.Variance = 3 borderRemoveCommandCallback.WhiteNoiseLength = 9 borderRemoveCommandCallback.Run(leadImage) End Sub Private Sub BorderRemoveCommand_BorderRemove_S1(ByVal sender As Object, ByVal e As BorderRemoveCommandEventArgs) Handles borderRemoveCommandCallback.BorderRemove Dim Border As String Select Case (e.Border) Case BorderRemoveBorderFlags.Top Border = "Top" Case BorderRemoveBorderFlags.Left Border = "Left" Case BorderRemoveBorderFlags.Right Border = "Right" Case BorderRemoveBorderFlags.Bottom Border = "Bottom" Case Else Border = "" End Select MessageBox.Show("Bounds ( " + Convert.ToString(e.BoundingRectangle.Left) + ", " + Convert.ToString(e.BoundingRectangle.Top) + ") - " + "( " + Convert.ToString(e.BoundingRectangle.Right) + ", " + Convert.ToString(e.BoundingRectangle.Bottom) + ")" + Chr(13) + " Border " + Border.ToString()) e.Status = RemoveStatus.Remove End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void BorderRemoveCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Clean.tif")); // Prepare the command BorderRemoveCommand command = new BorderRemoveCommand(); command.BorderRemove += new EventHandler<BorderRemoveCommandEventArgs>(command_BorderRemove_S1); command.Border = BorderRemoveBorderFlags.All; command.Flags = BorderRemoveCommandFlags.UseVariance; command.Percent = 20; command.Variance = 3; command.WhiteNoiseLength = 9; command.Run(image); } private void command_BorderRemove_S1(object sender, BorderRemoveCommandEventArgs e) { string Border; switch (e.Border) { case BorderRemoveBorderFlags.Top: Border = "Top"; break; case BorderRemoveBorderFlags.Left: Border = "Left"; break; case BorderRemoveBorderFlags.Right: Border = "Right"; break; case BorderRemoveBorderFlags.Bottom: Border = "Bottom"; break; default: Border = ""; break; } MessageBox.Show("Bounds " + "( " + e.BoundingRectangle.Left + ", " + e.BoundingRectangle.Top + ") - " + "( " + e.BoundingRectangle.Right + ", " + e.BoundingRectangle.Bottom + ")" + "\n Border " + Border.ToString()); e.Status = RemoveStatus.Remove; } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
public void BorderRemoveCommandExample(RasterImage image, Stream outStream) { // Prepare the command BorderRemoveCommand command = new BorderRemoveCommand(); command.BorderRemove += new EventHandler<BorderRemoveCommandEventArgs>(command_BorderRemove_S1); command.Border = BorderRemoveBorderFlags.All; command.Flags = BorderRemoveCommandFlags.UseVariance; command.Percent = 20; command.Variance = 3; command.WhiteNoiseLength = 9; command.Run(image); // Save result image RasterCodecs codecs = new RasterCodecs(); codecs.Save(image, outStream, RasterImageFormat.CcittGroup4, 1); image.Dispose(); } private void command_BorderRemove_S1(object sender, BorderRemoveCommandEventArgs e) { string Border; switch (e.Border) { case BorderRemoveBorderFlags.Top: Border = "Top"; break; case BorderRemoveBorderFlags.Left: Border = "Left"; break; case BorderRemoveBorderFlags.Right: Border = "Right"; break; case BorderRemoveBorderFlags.Bottom: Border = "Bottom"; break; default: Border = ""; break; } Debug.WriteLine("Bounds " + "( " + e.BoundingRectangle.Left + ", " + e.BoundingRectangle.Top + ") - " + "( " + e.BoundingRectangle.Right + ", " + e.BoundingRectangle.Bottom + ")" + "\n Border " + Border.ToString()); e.Status = RemoveStatus.Remove; } |
SilverlightVB | ![]() |
---|---|
Public Sub BorderRemoveCommandExample(ByVal image As RasterImage, ByVal outStream As Stream) ' Prepare the command Dim command As BorderRemoveCommand = New BorderRemoveCommand() AddHandler command.BorderRemove, AddressOf command_BorderRemove_S1 command.Border = BorderRemoveBorderFlags.All command.Flags = BorderRemoveCommandFlags.UseVariance command.Percent = 20 command.Variance = 3 command.WhiteNoiseLength = 9 command.Run(image) ' Save result image Dim codecs As RasterCodecs = New RasterCodecs() codecs.Save(image, outStream, RasterImageFormat.CcittGroup4, 1) image.Dispose() End Sub Private Sub command_BorderRemove_S1(ByVal sender As Object, ByVal e As BorderRemoveCommandEventArgs) Dim Border As String Select Case e.Border Case BorderRemoveBorderFlags.Top Border = "Top" Case BorderRemoveBorderFlags.Left Border = "Left" Case BorderRemoveBorderFlags.Right Border = "Right" Case BorderRemoveBorderFlags.Bottom Border = "Bottom" Case Else Border = "" End Select Debug.WriteLine("Bounds " & "( " & e.BoundingRectangle.Left & ", " & e.BoundingRectangle.Top & ") - " & "( " & e.BoundingRectangle.Right & ", " & e.BoundingRectangle.Bottom & ")" & Constants.vbLf & " Border " & Border.ToString()) e.Status = RemoveStatus.Remove End Sub |
- This command removes borders that commonly appear in scanned text documents. Any or all of the four borders can be detected and removed. The behavior of this command can be modified by using an Event Handler that handles the BorderRemoveCommandEventArgs.
- This command works only on 1-bit black and white images.
- If a region is selected, only the selected region will be changed by this command. If no region is selected, the whole image will be processed.
- This command does not support signed data images.
- This command does not support 32-bit grayscale images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Core.BorderRemoveCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
BorderRemoveCommand MembersLeadtools.ImageProcessing.Core Namespace
Cleaning Up 1-Bit Images
SmoothCommand Class
LineRemoveCommand Class
InvertedTextCommand Class
InvertedPageCommand Class
DotRemoveCommand Class
HolePunchRemoveCommand Class
HighQualityRotateCommand Class
MinimumCommand Class
MaximumCommand Class
Leadtools.ImageProcessing.Effects.RegionHolesRemovalCommand