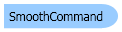
Visual Basic (Declaration) | |
---|---|
Public Class SmoothCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As SmoothCommand |
C# | |
---|---|
public class SmoothCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class SmoothCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Run the SmoothCommand on an image.
Visual Basic | ![]() |
---|---|
Public WithEvents smoothEventExample_S1 As SmoothCommand Public Sub SmoothCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Clean.tif")) ' Prepare the command smoothEventExample_S1 = New SmoothCommand smoothEventExample_S1.Flags = SmoothCommandFlags.FavorLong smoothEventExample_S1.Length = 2 smoothEventExample_S1.Run(leadImage) End Sub Private Sub SmoothEventExample_SmoothCommand_S1(ByVal sender As Object, ByVal e As SmoothCommandEventArgs) Handles smoothEventExample_S1.Smooth Dim BumpOrNeck As String Dim Direction As String If (e.BumpNick = SmoothCommandBumpNickType.Bump) Then BumpOrNeck = "Bump" Else BumpOrNeck = "Neck" End If If (e.Direction = SmoothCommandDirectionType.Horizontal) Then Direction = "Horizontal" Else Direction = "Vertical" End If MessageBox.Show("Type " + BumpOrNeck.ToString() + _ Chr(13) + " Row Column " + e.StartRow.ToString() + e.StartColumn.ToString() + _ Chr(13) + " Length " + e.Length.ToString() + _ Chr(13) + " Direction " + Direction.ToString()) e.Status = RemoveStatus.Remove End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void SmoothCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "clean.tif")); // Prepare the command SmoothCommand command = new SmoothCommand(); command.Smooth += new EventHandler<SmoothCommandEventArgs>(SmoothEventExample_S1); command.Flags = SmoothCommandFlags.FavorLong ; command.Length = 2; command.Run(image); } private void SmoothEventExample_S1(object sender, SmoothCommandEventArgs e) { string BumpOrNeck; string Direction; if (e.BumpNick == SmoothCommandBumpNickType.Bump) BumpOrNeck = "Bump"; else BumpOrNeck = "Neck"; if (e.Direction == SmoothCommandDirectionType.Horizontal) Direction = "Horizontal"; else Direction = "Vertical"; MessageBox.Show("Type " + BumpOrNeck + "\n Row Column " + e.StartRow.ToString() + e.StartColumn.ToString() + "\n Length " + e.Length + "\n Direction " + Direction); e.Status = RemoveStatus.Remove; } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
public void SmoothCommandExample(RasterImage image, Stream outStream) { // Prepare the command SmoothCommand command = new SmoothCommand(); command.Smooth += new EventHandler<SmoothCommandEventArgs>(SmoothEventExample_S1); command.Flags = SmoothCommandFlags.FavorLong; command.Length = 2; command.Run(image); // Save result image RasterCodecs codecs = new RasterCodecs(); codecs.Save(image, outStream, RasterImageFormat.CcittGroup4, 1); image.Dispose(); } private void SmoothEventExample_S1(object sender, SmoothCommandEventArgs e) { string BumpOrNeck; string Direction; if (e.BumpNick == SmoothCommandBumpNickType.Bump) BumpOrNeck = "Bump"; else BumpOrNeck = "Neck"; if (e.Direction == SmoothCommandDirectionType.Horizontal) Direction = "Horizontal"; else Direction = "Vertical"; Debug.WriteLine("Type " + BumpOrNeck + "\n Row Column " + e.StartRow.ToString() + e.StartColumn.ToString() + "\n Length " + e.Length + "\n Direction " + Direction); e.Status = RemoveStatus.Remove; } |
SilverlightVB | ![]() |
---|---|
Public Sub SmoothCommandExample(ByVal image As RasterImage, ByVal outStream As Stream) ' Prepare the command Dim command As SmoothCommand = New SmoothCommand() AddHandler command.Smooth, AddressOf SmoothEventExample_S1 command.Flags = SmoothCommandFlags.FavorLong command.Length = 2 command.Run(image) ' Save result image Dim codecs As RasterCodecs = New RasterCodecs() codecs.Save(image, outStream, RasterImageFormat.CcittGroup4, 1) image.Dispose() End Sub Private Sub SmoothEventExample_S1(ByVal sender As Object, ByVal e As SmoothCommandEventArgs) Dim BumpOrNeck As String Dim Direction As String If e.BumpNick = SmoothCommandBumpNickType.Bump Then BumpOrNeck = "Bump" Else BumpOrNeck = "Neck" End If If e.Direction = SmoothCommandDirectionType.Horizontal Then Direction = "Horizontal" Else Direction = "Vertical" End If Debug.WriteLine("Type " & BumpOrNeck & Constants.vbLf & " Row Column " & e.StartRow.ToString() & e.StartColumn.ToString() & Constants.vbLf & " Length " & e.Length + Constants.vbLf & " Direction " & Direction) e.Status = RemoveStatus.Remove End Sub |
- This command smooths the text in scanned text documents. The behavior of this command can be modified by using an Event Handler that handles the SmoothCommandEventArgs. This command works only on 1-bit black and white images.
- This command works only on 1-bit black and white images.
- If a region is selected, only the selected region will be changed by this command. If no region is selected, the whole image will be processed.
- This command does not support signed data images.
- This command does not support 32-bit grayscale images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Core.SmoothCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
SmoothCommand MembersLeadtools.ImageProcessing.Core Namespace
Cleaning Up 1-Bit Images
BorderRemoveCommand Class
LineRemoveCommand Class
InvertedTextCommand Class
InvertedPageCommand Class
DotRemoveCommand Class
HolePunchRemoveCommand Class
HighQualityRotateCommand Class
MinimumCommand Class
MaximumCommand Class
Leadtools.ImageProcessing.Effects.RegionHolesRemovalCommand