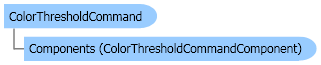
Visual Basic (Declaration) | |
---|---|
Public Class ColorThresholdCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As ColorThresholdCommand |
C# | |
---|---|
public class ColorThresholdCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class ColorThresholdCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Run the ColorThresholdCommand on an image.
Visual Basic | ![]() |
---|---|
Public Sub ColorThresholdCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")) ' Prepare the command Dim components() As ColorThresholdCommandComponent ReDim components(2) components(0) = New ColorThresholdCommandComponent components(1) = New ColorThresholdCommandComponent components(2) = New ColorThresholdCommandComponent components(0).MinimumRange = 128 components(0).MaximumRange = 255 components(0).Flags = 0 components(1).MinimumRange = 128 components(1).MaximumRange = 255 components(1).Flags = 0 components(2).MinimumRange = 128 components(2).MaximumRange = 255 components(2).Flags = 0 Dim command As ColorThresholdCommand = New ColorThresholdCommand(ColorThresholdCommandType.Rgb, components) 'Apply color Threshold effect on the image. command.Run(leadImage) codecs.Save(leadImage, Path.Combine(LEAD_VARS.ImagesDir, "Result.jpg"), RasterImageFormat.Jpeg, 24) End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void ColorThresholdCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")); // Prepare the command ColorThresholdCommandComponent[] Components = new ColorThresholdCommandComponent[3]; Components[0] = new ColorThresholdCommandComponent(); Components[0].MinimumRange = 128; Components[0].MaximumRange = 255; Components[0].Flags = 0; Components[1] = new ColorThresholdCommandComponent(); Components[1].MinimumRange = 128; Components[1].MaximumRange = 255; Components[1].Flags = 0; Components[2] = new ColorThresholdCommandComponent(); Components[2].MinimumRange = 128; Components[2].MaximumRange = 255; Components[2].Flags = 0; ColorThresholdCommand command = new ColorThresholdCommand(ColorThresholdCommandType.Rgb, Components); //Apply color Threshold effect on the image. command.Run(image); codecs.Save(image, Path.Combine(LEAD_VARS.ImagesDir, "Result.jpg"), RasterImageFormat.Jpeg, 24); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
public void ColorThresholdCommandExample(RasterImage image, Stream outStream) { // Prepare the command ColorThresholdCommandComponent[] Components = new ColorThresholdCommandComponent[3]; Components[0] = new ColorThresholdCommandComponent(); Components[0].MinimumRange = 128; Components[0].MaximumRange = 255; Components[0].Flags = 0; Components[1] = new ColorThresholdCommandComponent(); Components[1].MinimumRange = 128; Components[1].MaximumRange = 255; Components[1].Flags = 0; Components[2] = new ColorThresholdCommandComponent(); Components[2].MinimumRange = 128; Components[2].MaximumRange = 255; Components[2].Flags = 0; ColorThresholdCommand command = new ColorThresholdCommand(ColorThresholdCommandType.Rgb, Components); //Apply color Threshold effect on the image. command.Run(image); // Save result image RasterCodecs codecs = new RasterCodecs(); codecs.Save(image, outStream, RasterImageFormat.Jpeg, 24); image.Dispose(); } |
SilverlightVB | ![]() |
---|---|
Public Sub ColorThresholdCommandExample(ByVal image As RasterImage, ByVal outStream As Stream) ' Prepare the command Dim Components As ColorThresholdCommandComponent() = New ColorThresholdCommandComponent(2){} Components(0) = New ColorThresholdCommandComponent() Components(0).MinimumRange = 128 Components(0).MaximumRange = 255 Components(0).Flags = 0 Components(1) = New ColorThresholdCommandComponent() Components(1).MinimumRange = 128 Components(1).MaximumRange = 255 Components(1).Flags = 0 Components(2) = New ColorThresholdCommandComponent() Components(2).MinimumRange = 128 Components(2).MaximumRange = 255 Components(2).Flags = 0 Dim command As ColorThresholdCommand = New ColorThresholdCommand(ColorThresholdCommandType.Rgb, Components) 'Apply color Threshold effect on the image. command.Run(image) ' Save result image Dim codecs As RasterCodecs = New RasterCodecs() codecs.Save(image, outStream, RasterImageFormat.Jpeg, 24) image.Dispose() End Sub |
Every color space component may have a different range:
This class works as follows:
For more information, refer to Introduction to Image Processing With LEADTOOLS.
For more information, refer to Correcting Colors.
Color Component | Range for 8 bit per component. | Range for 16 bit per component. |
RGB | ||
R | 0 ... 255 | 0 ... 65535 |
G | 0 ... 255 | 0 ... 65535 |
B | 0 ... 255 | 0 ... 65535 |
HSV | ||
H | 0 ... 360 | 0 ... 36000 |
S | 0 ... 100 | 0 ... 10000 |
V | 0 ... 255 | 0 ... 65535 |
HLS | ||
H | 0 ... 360 | 0 ... 36000 |
L | 0 ... 255 | 0 ... 65535 |
S | 0 ... 100 | 0 ... 10000 |
XYZ | ||
X | 0 ... 255 | 0 ... 65535 |
Y | 0 ... 255 | 0 ... 65535 |
Z | 0 ... 255 | 0 ... 65535 |
YCrCb | ||
Y | 0 ... 255 | 0 ... 65535 |
Cr | -128 ... 127 | -32768 ... 32767 |
Cb | -128 ... 127 | -32768 ... 32767 |
YUV | ||
Y | 0 ... 255 | 0 ... 65535 |
U | -112 ... 111 | -28567 ... 28566 |
V | -138 ... 137 | -35337 ... 35336 |
LAB | ||
L | 0 ... 100 | 0 ... 10000 |
A | -128 ... 127 | -32768 ... 32767 |
B | -128 ... 127 | -32768 ... 32767 |
CMY | ||
C | 0 ... 255 | 0 ... 65535 |
M | 0 ... 255 | 0 ... 65535 |
Y | 0 ... 255 | 0 ... 65535 |
This class works as follows:
- The image is converted to the required color space.
- For every pixel, the following operations are performed: Each component is compared with the MinimumRange and MaximumRange values of the appropriate ColorThresholdCommandComponent class.
- If it is inside the range, the component is considered to have "passed" the test.
- If it is outside the range, the component has been rejected". The ColorThresholdCommandFlags.BandReject flag inverts this by making "rejected" components "passed" and vice-versa.
- If ColorThresholdCommandFlags.EffectChannel is set, the components are modified independently. If ColorThresholdCommandFlags.EffectAll has been set, the pixel is rejected by the test if any component is rejected. If the pixel/component is rejected, then:
- If ColorThresholdCommandFlags.SetToMinimum is set, the rejected pixel/component is set to 0.
- If ColorThresholdCommandFlags.SetToMaximum is set, the rejected pixel/component is set to 255.
- If ColorThresholdCommandFlags.SetToClamp is set, components less that MinimumRange go to 0 and components greater than MaximumRange go to 255. This would work only with the ColorThresholdCommandFlags.EffectChannel and ColorThresholdCommandFlags.BandBass flags.
- This class works for 1, 2, 3, _ 8,16, 24, 32, 48 and 64-bit color images and can support regions for 24 and 48-bit images. If a 24 or 48-bit image has a region the effect will be applied on the region only.
- One class is used for each color component. The order is considered to be the same as in the xxx Space name. For example, for Rgb Space:
- Components[0] class is used for the Red component.
- Components[1] class is used for the Green component.
- Components[2] class is used for the Blue component.
- This command does not support 32-bit grayscale images.
- This command does not support signed images.
For more information, refer to Introduction to Image Processing With LEADTOOLS.
For more information, refer to Correcting Colors.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Color.ColorThresholdCommand
Target Platforms: Silverlight, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
ColorThresholdCommand MembersLeadtools.ImageProcessing.Color Namespace
Introduction to Image Processing With LEADTOOLS
Correcting Colors
Leadtools.ImageProcessing.Effects.BinaryFilterCommand
ColorSeparateCommand Class
ColorMergeCommand Class
Leadtools.ImageProcessing.Effects.AddNoiseCommand
IntensityDetectCommand Class
Leadtools.ImageProcessing.Effects.SpatialFilterCommand
Leadtools.ImageProcessing.Effects.BinaryFilterCommand
Leadtools.ImageProcessing.Core.MaximumCommand
Leadtools.ImageProcessing.Core.MinimumCommand
Leadtools.ImageProcessing.SpecialEffects.ShadowCommand
ChangeHueSaturationIntensityCommand Class
ColorThresholdCommand Class
Leadtools.ImageProcessing.Core.DiscreteFourierTransformCommand
Leadtools.ImageProcessing.Core.FastFourierTransformCommand
Leadtools.ImageProcessing.Core.FourierTransformDisplayCommand
Leadtools.ImageProcessing.Core.FrequencyFilterCommand
Leadtools.ImageProcessing.Core.FrequencyFilterMaskCommand
Leadtools.ImageProcessing.Effects.DirectionEdgeStatisticalCommand
MathematicalFunctionCommand Class
Leadtools.ImageProcessing.SpecialEffects.RevEffectCommand
SegmentCommand Class
Leadtools.ImageProcessing.Core.SubtractBackgroundCommand
Leadtools.ImageProcessing.Effects.UserFilterCommand
AdjustTintCommand Class
Leadtools.ImageProcessing.Core.HalfToneCommand