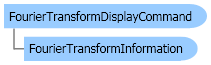
Visual Basic (Declaration) | |
---|---|
Public Class FourierTransformDisplayCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As FourierTransformDisplayCommand |
C# | |
---|---|
public class FourierTransformDisplayCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class FourierTransformDisplayCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Runs the Leadtools.ImageProcessing.Core.FourierTransformDisplayCommand on an image, applies the Discrete Fourier Transform command to it, and displays the data.
Visual Basic | ![]() |
---|---|
Public Sub FourierTransformDisplayCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")) ' Prepare the command Dim FTArray As FourierTransformInformation = New FourierTransformInformation(leadImage) Dim rcRange As LeadRect = New LeadRect(0, 0, leadImage.Width - 1, leadImage.Height - 1) Dim DFTcommand As DiscreteFourierTransformCommand = New DiscreteFourierTransformCommand Dim command As FourierTransformDisplayCommand = New FourierTransformDisplayCommand DFTcommand.FourierTransformInformation = FTArray DFTcommand.Range = rcRange DFTcommand.Flags = DiscreteFourierTransformCommandFlags.DiscreteFourierTransform _ Or DiscreteFourierTransformCommandFlags.Gray _ Or DiscreteFourierTransformCommandFlags.Range _ Or DiscreteFourierTransformCommandFlags.InsideX _ Or DiscreteFourierTransformCommandFlags.OutsideY ' apply DFT DFTcommand.Run(leadImage) command.Flags = FourierTransformDisplayCommandFlags.Log Or FourierTransformDisplayCommandFlags.Magnitude command.FourierTransformInformation = FTArray 'plot frequency magnitude command.Run(leadImage) End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void FourierTransformDisplayCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")); // Prepare the command FourierTransformInformation FTArray = new FourierTransformInformation(image); LeadRect rcRange = new LeadRect(0, 0, image.Width - 1, image.Height - 1); DiscreteFourierTransformCommand DFTcommand = new DiscreteFourierTransformCommand(); FourierTransformDisplayCommand command = new FourierTransformDisplayCommand(); DFTcommand.FourierTransformInformation = FTArray; DFTcommand.Range = rcRange; DFTcommand.Flags = DiscreteFourierTransformCommandFlags.DiscreteFourierTransform | DiscreteFourierTransformCommandFlags.Gray | DiscreteFourierTransformCommandFlags.Range | DiscreteFourierTransformCommandFlags.InsideX | DiscreteFourierTransformCommandFlags.InsideY; // apply DFT DFTcommand.Run(image); command.Flags = FourierTransformDisplayCommandFlags.Log | FourierTransformDisplayCommandFlags.Magnitude; command.FourierTransformInformation = FTArray; //plot frequency magnitude. command.Run(image); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
SilverlightVB | ![]() |
---|---|
- This command converts the Data property of the FourierTransformInformation object computed by FastFourierTransformCommand or FastFourierTransformCommand commands into an image. The resulting image may be displayed such that it shows either the frequency harmonics amplitude data or phase data. The (0,0) frequency is located in the image center, positive X harmonics in the right half and positive Y harmonics located in the lower half of the image.
- This command does not work on regions. If the image has a region the command ignores it and processes the entire image.
- The Run method image should be allocated prior to calling this command. The size of the image must be the same size as the image that was used to generate the information in the Data property of the FourierTransformInformation object.
- This command does not support 12 and 16-bit grayscale and 48 and 64-bit color images. If the image is 12 and 16-bit grayscale and 48 and 64-bit color, the command will not threw an exception.
- This command does not support signed data images.
- This command does not support 32-bit grayscale images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Core.FourierTransformDisplayCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
FourierTransformDisplayCommand MembersLeadtools.ImageProcessing.Core Namespace
Removing Noise
DiscreteFourierTransformCommand Class
FrequencyFilterCommand Class
Leadtools.ImageProcessing.Effects.AverageCommand
MedianCommand Class
Leadtools.ImageProcessing.Effects.SpatialFilterCommand
Leadtools.ImageProcessing.Effects.BinaryFilterCommand
MinimumCommand Class
Leadtools.ImageProcessing.Effects.AddNoiseCommand
Leadtools.ImageProcessing.Color.IntensityDetectCommand
MaximumCommand Class
Leadtools.ImageProcessing.Color.ChangeHueSaturationIntensityCommand
Leadtools.ImageProcessing.Color.ColorThresholdCommand
Leadtools.ImageProcessing.Effects.DirectionEdgeStatisticalCommand
FastFourierTransformCommand Class
FourierTransformDisplayCommand Class
Leadtools.ImageProcessing.Effects.StatisticsInformationCommand
Leadtools.ImageProcessing.Effects.FeretsDiameterCommand
Leadtools.ImageProcessing.Effects.ObjectInformationCommand
Leadtools.ImageProcessing.Effects.RegionContourPointsCommand
Leadtools.ImageProcessing.Color.MathematicalFunctionCommand
Leadtools.ImageProcessing.SpecialEffects.RevEffectCommand
Leadtools.ImageProcessing.Color.SegmentCommand
SubtractBackgroundCommand Class
Leadtools.ImageProcessing.Effects.UserFilterCommand
Leadtools.ImageProcessing.SpecialEffects.FragmentCommand
Leadtools.ImageProcessing.Effects.HighPassCommand
Leadtools.ImageProcessing.Effects.UnsharpMaskCommand