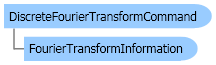
Visual Basic (Declaration) | |
---|---|
Public Class DiscreteFourierTransformCommand Inherits Leadtools.ImageProcessing.RasterCommand Implements IRasterCommand |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As DiscreteFourierTransformCommand |
C# | |
---|---|
public class DiscreteFourierTransformCommand : Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
C++/CLI | |
---|---|
public ref class DiscreteFourierTransformCommand : public Leadtools.ImageProcessing.RasterCommand, IRasterCommand |
Run the DiscreteFourierTransformCommand on an image.
Visual Basic | ![]() |
---|---|
Public Sub DiscreteFourierTransformCommandExample() Dim codecs As New RasterCodecs() codecs.ThrowExceptionsOnInvalidImages = True Dim leadImage As RasterImage = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")) ' Prepare the command Dim FTArray As FourierTransformInformation = New FourierTransformInformation(leadImage) Dim rcRange As LeadRect = New LeadRect(0, 0, leadImage.Width - 1, leadImage.Height - 1) Dim command As DiscreteFourierTransformCommand = New DiscreteFourierTransformCommand command.FourierTransformInformation = FTArray command.Range = rcRange command.Flags = DiscreteFourierTransformCommandFlags.DiscreteFourierTransform Or _ DiscreteFourierTransformCommandFlags.Gray Or _ DiscreteFourierTransformCommandFlags.Range Or _ DiscreteFourierTransformCommandFlags.InsideX Or _ DiscreteFourierTransformCommandFlags.InsideY 'Apply DFT. command.Run(leadImage) Dim disCommand As FourierTransformDisplayCommand = New FourierTransformDisplayCommand disCommand.Flags = FourierTransformDisplayCommandFlags.Log Or FourierTransformDisplayCommandFlags.Magnitude disCommand.FourierTransformInformation = command.FourierTransformInformation 'plot frequency magnitude disCommand.Run(leadImage) End Sub Public NotInheritable Class LEAD_VARS Public Const ImagesDir As String = "C:\Users\Public\Documents\LEADTOOLS Images" End Class |
C# | ![]() |
---|---|
public void DiscreteFourierTransformCommandExample() { // Load an image RasterCodecs codecs = new RasterCodecs(); codecs.ThrowExceptionsOnInvalidImages = true; RasterImage image = codecs.Load(Path.Combine(LEAD_VARS.ImagesDir, "Master.jpg")); // Prepare the command FourierTransformInformation FTArray = new FourierTransformInformation(image); LeadRect rcRange = new LeadRect(0, 0, image.Width - 1, image.Height - 1); DiscreteFourierTransformCommand command = new DiscreteFourierTransformCommand(); command.FourierTransformInformation = FTArray; command.Range = rcRange; command.Flags = DiscreteFourierTransformCommandFlags.DiscreteFourierTransform | DiscreteFourierTransformCommandFlags.Gray | DiscreteFourierTransformCommandFlags.Range | DiscreteFourierTransformCommandFlags.InsideX | DiscreteFourierTransformCommandFlags.InsideY; //Apply DFT. FourierTransformDisplayCommand disCommand = new FourierTransformDisplayCommand(); disCommand.Flags = FourierTransformDisplayCommandFlags.Log| FourierTransformDisplayCommandFlags.Magnitude; disCommand.FourierTransformInformation = command.FourierTransformInformation; // plot frequency magnitude disCommand.Run(image); } static class LEAD_VARS { public const string ImagesDir = @"C:\Users\Public\Documents\LEADTOOLS Images"; } |
SilverlightCSharp | ![]() |
---|---|
SilverlightVB | ![]() |
---|---|
- This command converts the image from the time domain to the frequency domain and vice versa using the Discrete Fourier Transform algorithm. Use the FastFourierTransformCommand to use a Fast Fourier Transform algorithm on an image. Please note however, that this command does not impose the size restrictions (the width and height having to be powers of 2) that the Fast Fourier Transform method, FastFourierTransformCommand, imposes upon images.
- Before using this command, you need to construct an instance from FourierTransformInformation.
- The image can be transformed back into the original image minus the noise using the InverseDiscreteFourierTransform
- This command does not work on regions. If the image has a region the command ignores it and processes the entire image.
- This command does not support 12 and 16-bit grayscale and 48 and 64-bit color images. If the image is 12 and 16-bit grayscale and 48 and 64-bit color, the method will not threw an exception.
- This command does not support 32-bit grayscale images.
- This command does not support signed images.
System.Object
Leadtools.ImageProcessing.RasterCommand
Leadtools.ImageProcessing.Core.DiscreteFourierTransformCommand
Target Platforms: Silverlight 3.0, Windows XP, Windows Server 2003 family, Windows Server 2008 family, Windows Vista, Windows 7, MAC OS/X (Intel Only)
Reference
DiscreteFourierTransformCommand MembersLeadtools.ImageProcessing.Core Namespace
Removing Noise
FastFourierTransformCommand Class
FrequencyFilterCommand Class
FourierTransformDisplayCommand Class
Leadtools.ImageProcessing.Effects.AverageCommand
MedianCommand Class
Leadtools.ImageProcessing.Effects.AddNoiseCommand
Leadtools.ImageProcessing.Color.IntensityDetectCommand
Leadtools.ImageProcessing.Effects.SpatialFilterCommand
Leadtools.ImageProcessing.Effects.BinaryFilterCommand
MaximumCommand Class
MinimumCommand Class
Leadtools.ImageProcessing.Color.ChangeHueSaturationIntensityCommand
Leadtools.ImageProcessing.Color.ColorThresholdCommand
DiscreteFourierTransformCommand Class
Leadtools.ImageProcessing.Effects.DirectionEdgeStatisticalCommand
Leadtools.ImageProcessing.Effects.StatisticsInformationCommand
Leadtools.ImageProcessing.Effects.FeretsDiameterCommand
Leadtools.ImageProcessing.Effects.ObjectInformationCommand
Leadtools.ImageProcessing.Effects.RegionContourPointsCommand
Leadtools.ImageProcessing.Color.MathematicalFunctionCommand
Leadtools.ImageProcessing.SpecialEffects.RevEffectCommand
Leadtools.ImageProcessing.Color.SegmentCommand
SubtractBackgroundCommand Class
Leadtools.ImageProcessing.Effects.UserFilterCommand
Leadtools.ImageProcessing.SpecialEffects.FragmentCommand
Leadtools.ImageProcessing.Effects.HighPassCommand
Leadtools.ImageProcessing.Effects.UnsharpMaskCommand